DOM Node Selection with JavaScript
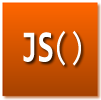
Selecting a Single Unique Tag
The simplest situation is when you need to select a single unique tag from the page. You can do this by giving your tag an id attribute, and then using document.getElementById(). See below.
<div id="MyDiv"></div>
<script>
var MyDiv = document.getElementById('MyDiv');
</script>
A couple of notes about this; first of all, never ever assign the same id to multiple tags. This goes completely against what the id attribute is for-to uniquely identify a single tag. Secondly, it real-world scenarios, you're most likely to have your JavaScript in the head of the document, or included from the head of your document.
Selecting a Group of Tags with the Same Name
What if you wanted to select a group of tags though? What if, say, you wanted to grab all div tags on your page? You can do this easily enough by using getElementsByTagName(). See below.
<div id="MyDiv">
<span id="MySpan"></span>
<span id="Span2"></span>
</div>
<script>
var MyDiv = document.getElementById('MyDiv');
var SpanList = MyDiv.getElementsByTagName('span');
</script>
One thing you'll notice from the above is that it differs in it's use from getElementById(). Instead of just being a method on the document, getElementsByTagName() is a method built into all DOM elements. It can be called on any DOM element, which will search all of its descendants for a match. Also, instead of returning a single tag object, it returns an array of matches.
Selecting a Group of Tags
In the examples we've encountered so far, we've been able to select a single tag, and select a group of tags (as long as those tags had the same tag name). But what if we wanted to select a group of tags that had entirely different names? In that case, neither getElementById() or getElementsByTagName() are sufficient. Enter getElementsByClassName().
<div class="target">
<span id="target"></span>
<span id="target"></span>
</div>
<script>
var TagList = document.getElementsByClassName('target');
</script>
Similar to getElementsByTagName(), getElementsByClassName() is a method that can be called on any DOM node, and returns an array of elements. Compared to our first two methods, getElementsByClassName() is a relative newcomer; although its been supported in most browsers for some time, it only made it's debut in Internet Explorer in version 9.







No comments :
Post a Comment